Now a days many ASP.NET applications use jQuery Ajax in one or the other way. jQuery methods such as $.ajax(), $.get() and $.post() are commonly used in such applications. When you make multiple Ajax calls from a single page you may take advantage of the global Ajax event handler methods of jQuery. To that end this article provides a quick reference of various global Ajax configuration methods that can simplify your jQuery code.
As an example you will call the following ASP.NET MVC actions from jQuery code:
public ActionResult GetData()
{
System.Threading.Thread.Sleep(4000);
return Json($"Server timestamp : {DateTime.Now}");
}
The GetData() action simply asks the current thread to sleep for 4 seconds and then returns server side date-time stamp to the caller.
To invoke the above action you can use $.ajax() method as shown below:
$.ajax({
url: "/home/getdata",
type: "GET",
dataType: "json",
success: function (msg) {
$("#response").html(msg);
}
});
The success callback simply displays the timestamp returned from the server into a response <div> element. It is also assumed that the page contains an animated GIF image with ID of progress.
Ok. Now, let's use some global Ajax event handler methods of jQuery to configure things such as progress indicator and error message.
.ajaxSend()
The ajaxSend() method is used to execute some code just before making an Ajax request. You can use this method to display a common progress indicator to all the Ajax requests arising from a page. The following code shows how .ajaxSend() can be used:
$(document).ajaxSend(function () {
$("#progress").show();
});
As you can see, the ajaxSend() method is called on the document selector and takes a callback function. The callback function is triggered just before making each Ajax request from the page. For example, if a page makes three Ajax requests, the callback function gets executed thrice. In this case the callback function simply shows a progress indicator in the form of an animated GIF.
The following figure shows a sample run of the above code.
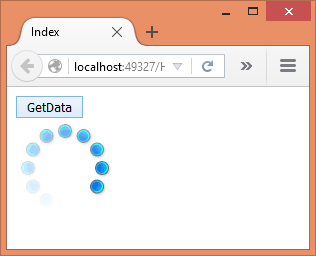
.ajaxComplete()
The ajaxComplete() method is used to execute some code when an Ajax request completes - successfully or with error. You can use this method to hide the progress indicator shown using the ajaxSend() method. The following code shows the usage of this method:
$(document).ajaxComplete(function () {
$("#progress").hide();
});
Just like ajaxSend(), the ajaxComplete() method is also called on the document selector. In this case the callback function simply hides the progress indicator.
.ajaxStart()
The callbacks registered through the ajaxSend() and ajaxComplete() methods are called for each and every Ajax request being invoked from the page. This is fine when your code makes only one Ajax request at a time. Imagine a case where you have multiple Ajax requests being made at the same time (or within a same time window). If you use ajaxSend() in this case, the show() method will be called even if the progress indicator is already visible from the previous call. You can correct the behavior if you show the progress indicator only when the first ever Ajax request is made. That's where ajaxStart() method comes handy.
$(document).ajaxStart(function () {
$("#progress").show();
});
When an Ajax request is being sent, jQuery checks whether there are any other pending requests from the page. If there are no pending Ajax requests the ajaxStart() callback is triggered.
.ajaxStop()
The counterpart of ajaxStart() is ajaxStop(). The callback registered through ajaxStop() is called whenever all the Ajax requests complete. When an Ajax request completes jQuery checks whether there are any other pending requests. If there are no pending requests jQuery triggers the ajaxStop() callback. The following code shows its usage:
$(document).ajaxStop(function () {
$("#progress").hide();
});
.ajaxSuccess()
The ajaxSuccess() method is used to perform some work when an Ajax request completes successfully. Notice the difference between ajaxComplete() and ajaxSuccess(). The ajaxComplete() callback is triggered irrespective of whether an Ajax request was successful or not. The ajaxSuccess(), on the other hand, is called only if an Ajax request is successful.
$(document).ajaxSuccess(function () {
$("#status").html(
"The Ajax request was completed successfully!");
});
.ajaxError()
The ajaxError() method is used to perform some work when an Ajax request completes with some error. You may use this method to display error indicator or to do some cleanup.
$(document).ajaxError(function () {
$("#status").html(
"There was an error while executing the Ajax request!");
});
Summary
- ajaxSend() triggers a registered callback every time an Ajax request is sent.
- ajaxComplete() triggers a registered callback every time an Ajax request completes.
- ajaxSuccess() triggers a registered callback every time an Ajax request completes successfully.
- ajaxError() triggeres a registered callback every time an Ajax request completes with an error.
- ajaxStart() triggers a registered callback when the first Ajax request begins.
- ajaxStop() triggers a registered callback when all the Ajax requests complete.