Web API allows you to create RESTful services easily. A typical Web API implements GET, POST, PUT and DELETE verbs by writing methods intended to handle each of these HTTP verbs. Although the actual method implementation can be anything as per your application requirement, you need to follow certain method signatures. For example, consider the following methods:
public void Post(MyClass data)
{
//do something with emp and cust here.
}
public void Put(int id, MyClass data)
{
//do something here.
}
The above code shows the template for Post() and Put() methods. The Post() method signature accepts the data accompanying the request as MyClass object. You can't have multiple parameters to Post() method. On the same lines the Put() method accepts the request data as the second parameter. Here again you can't have more parameters to receive the data. In short, a request to Web API wraps the data accompanying the request in one single object.
At times, however, you need to pass multiple independent pieces of information to the Web API. This poses a limitation to developers. You can overcome this problem in two ways:
- Accept data as array or ArrayList of objects.
- Create a class that wraps all the individual pieces and receive data as an instance of that class.
Let's see how these approaches look like at code level. Suppose you have two classes - Employee and Customer - as shown below:
public class Employee
{
public int EmployeeID { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
public class Customer
{
public string CustomerID { get; set; }
public string CompanyName { get; set; }
}
The Employee class has three properties namely EmployeeID, FirstName and LastName. The Customer class has two properties namely CustomerID and CompanyName. Now assume that you will be calling a Web API to store an Employee and a Customer into the database. In this example, let's assume you will be using jQuery to call the Web API (the approach remains the same even with HttpClient).
Next, consider the following Web API method:
public void Post(object[] data)
{
Employee emp = JsonConvert.DeserializeObject<Employee>(data[0].ToString());
Customer cust = JsonConvert.DeserializeObject<Customer>(data[1].ToString());
//do something with emp and cust here.
}
As you can see the Post() method has one parameter of type object array. If you want you can also use ArrayList instead of plain array. Inside, the code uses Json.NET to deserialize individual objects into their respective types. Of course, in this case you must ensure that the object array contains the objects in a specific sequence. In this example, Employee object must be available at index 0 and Customer object at index 1. Once you get the Employee and Customer objects you can store them into a database or process them any way you want.
The client side jQuery code that calls this Web API method is as follows:
$(document).ready(function () {
var objEmployee = {};
objEmployee.EmployeeID = 1;
objEmployee.FirstName = "Nancy";
objEmployee.LastName = "Davolio";
var objCustomer = {};
objCustomer.CustomerID = "ALFKI";
objCustomer.CompanyName = "Alfreds Futterkiste";
var strFinal = "[" + JSON.stringify(objEmployee) + "," +
JSON.stringify(objCustomer) + "]";
var options = {};
options.url = "/api/Values";
options.type = "POST";
options.data = strFinal;
options.dataType = "json";
options.contentType = "application/json";
options.success = function () { alert("Success"); };
options.error = function () { alert("Error"); };
$.ajax(options);
});
The above jQuery code creates a JavaScript object that mimics the Employee class on the server. It sets the EmployeeID, FirstName and LastName properties of the object to 1, Nancy and Davolio respectively. On the same lines another JavaScript object is created that mimics the Customer object on the server. The CustomerID and CompanyName properties of this object are set to ALFKI and Alfreds Futterkiste respectively. Then these two JavaScript objects are combined to form an array in JSON format. The strFinal variable holds this array. A sample array looks like this:
[
{
"EmployeeID" : 1,
"FirstName" : "Nancy",
"LastName" : "Davolio"
}
,
{
"CustomerID" : "ALFKI",
"CompanyName" : "Alfreds Futterkiste"
}
]
Then an Ajax call is made to the Web API. The options object provides all the settings required to make the call. The type property of the options object points to the Web API (/api/Values) in this case. The type property indicates the type of request (POST in this case). The data property is set to the strFinal variable. The dataType and contentType properties are set to json and application/json respectively. The success and error properties simply point to functions that display a message using an alert dialog. Finally, jQuery $.ajax() is used to invoke the Web API.
To test this code, run the application after setting a breakpoint in the Post() method. The following figure shows how the data is received in the object array:
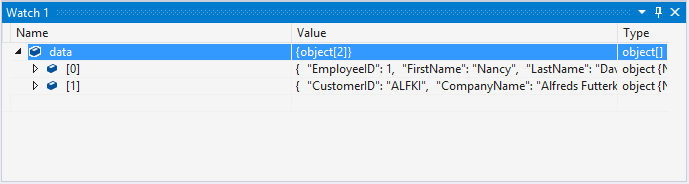
As you can see the data array has two elements. Each element is of type string. The string represents the JSON representation of the respective object.
In the second approach you can wrap the Employee and Customer objects into another class, say EmployeeCustomer, as shown below:
public class EmployeeCustomer
{
public Employee EmployeeData { get; set; }
public Customer CustomerData { get; set; }
}
The EmployeeCustomer class has two properties - EmployeeData and CustomerData - that are of type Employee and Customer respectively. The Post() method signature is like this:
public void Post(EmployeeCustomer data)
{
Employee emp = data.EmployeeData;
Customer cust = data.CustomerData;
//do something with emp and cust here.
}
In this case Post() accepts a parameter of type EmployeeCustomer. To call this Web API method from the client side you can use the following jQuery code:
$(document).ready(function () {
var objEmployee = {};
objEmployee.EmployeeID = 1;
objEmployee.FirstName = "Nancy";
objEmployee.LastName = "Davolio";
var objCustomer = {};
objCustomer.CustomerID = "ALFKI";
objCustomer.CompanyName = "Alfreds Futterkiste";
var strFinal = JSON.stringify({
EmployeeData: objEmployee,
CustomerData: objCustomer });
var options = {};
options.url = "/api/Values";
options.type = "POST";
options.data = strFinal;
options.dataType = "json";
options.contentType = "application/json";
options.success = function () { alert("Success"); };
options.error = function () { alert("Success"); };
$.ajax(options);
});
Most of the code shown above is identical to the previous example except the line marked in bold letters. This time you create a JavaScript object with two properties - EmployeeData and CustomerData. You set these properties to the objEmployee and objCustomer respectively. The strFinal variable contains the JSON representation of this JavaScript object.
When you run the above code after setting a breakpoint at the Post() method you will see the EmployeeCustomer object as follows:
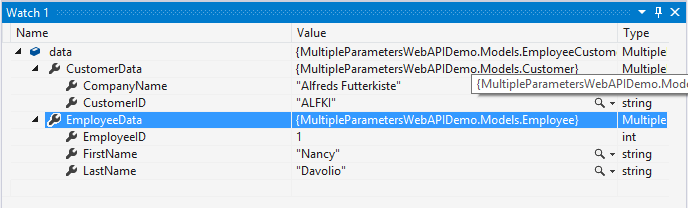
As you can see the EmployeeData and CustomerData properties of the EmployeeCustomer object are populated as expected.