In the previous article you learnt to create a Web API using ASP.NET Core. A Web API can be consumed by local clients or remote clients. Local clients are the clients that are housed in the same web application as the Web API. Remote clients are the clients that are not part of the Web API application.
As far as web applications are concerned a typical local client takes a form of jQuery (or JavaScript) Ajax driven user interface that consumes the Web API. For example, consider a simple page shown below:
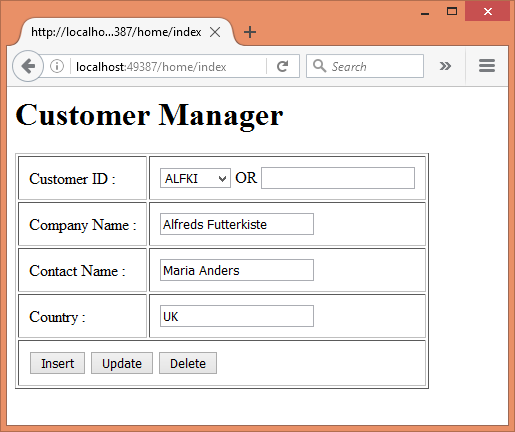
The above client to the CustomerService Web API consists of a dropdown list and four textboxes. The dropdown list contains a list of existing CustomerIDs. Selecting a CustomerID populates the other textboxes with the respective values. You can then modify the values and click on Update button to save the changes. To insert a new Customer, specify its CustomerID in the textbox besides the dropdown list, fill other details and hit the Insert button. Finally, to delete a Customer, select its CustomerID from the dropdown list and click on the Delete button.
To create this page, add a new controller - HomeController - in the Controllers folder. Also add Index view and write the following HTML markup into it:
<h1>Customer Manager</h1>
<form>
<table border="1">
<tr>
<td>Customer ID :</td>
<td>
<select id="customerid"></select>
OR
<input id="newcustomerid" type="text" />
</td>
</tr>
<tr>
<td>Company Name :</td>
<td><input id="companyname" type="text" /></td>
</tr>
<tr>
<td>Contact Name :</td>
<td><input id="contactname" type="text" /></td>
</tr>
<tr>
<td>Country :</td>
<td><input id="country" type="text" /></td>
</tr>
<tr>
<td colspan="2">
<input type="button" id="insert"
value="Insert" />
<input type="button" id="update"
value="Update" />
<input type="button" id="delete"
value="Delete" />
</td>
</tr>
</table>
<br />
<div id="msg"></div>
</form>
To invoke the Web API you created earlier, you will use jQuery Ajax. So, add Scripts folder under the wwwroot folder and place jQuery library into it.
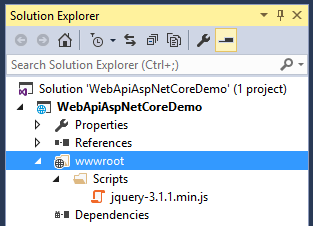
Also, add a <script> reference to the jQuery library in the <head> section of the Index view.
<script src="~/Scripts/jquery-3.1.1.min.js"></script>
There are in all five jQuery Ajax calls needed by our client. They are as follows:
- As soon as the page loads in the browser, the dropdown list should be filled with all the existing CustomerIDs. This is done by calling the Get() Web API action through jQuery Ajax.
- When you pick a CustomerID from the dropdown list its details such as CompanyName, ContactName and Country are displayed in the respective textboxes. This is done by calling the Get(id) Web API action from the change event handler of the dropdown list.
- Clicking Insert, Update and Delete buttons call the Post(), Put() and Delete() Web API actions respectively, again through jQuery Ajax.
Ok. Let's go ahead and write the first Ajax call.
Filling the dropdown list with CustomerIDs
$(document).ready(function () {
var options = {};
options.url = "/api/customerservice";
options.type = "GET";
options.dataType = "json";
options.success = function (data) {
data.forEach(function (element) {
$("#customerid").append("<option>"
+ element.customerID + "</option>");
});
};
options.error = function () {
$("#msg").html("Error while
calling the Web API!");
};
$.ajax(options);
});
The above code makes an Ajax request using $.ajax() method of jQuery. Notice how the url, type and dataType properties of the options object are specified. Since we wish to invoke Get() action, the url points to the Web API end point. The HTTP verb used is GET and the response data type is set to json.
The success function simply fills the dropdown list with a series of <option> element each wrapping a CustoemrID. The error function displays an error message in case something goes wrong while calling the Web API.
Displaying details of a selected customer
The change event handler of the dropdown looks like this:
$("#customerid").change(function () {
var options = {};
options.url = "/api/customerservice/" +
$("#customerid").val();
options.type = "GET";
options.dataType = "json";
options.success = function (data) {
$("#companyname").val(data.companyName);
$("#contactname").val(data.contactName);
$("#country").val(data.country);
};
options.error = function (a, b, c) {
alert(a.responseText);
$("#msg").html("Error while
calling the Web API!");
};
$.ajax(options);
});
This code is quite similar to the previous one. However, it appends the CustomerID whose details are to be fetched to the url. The success function fills the three textboxes with CompanyName, ContactName and Country. Notice something important - the property names are automatically converted to use camel casing. This way client side code gets to stick with the JavaScript ways of naming the things whereas server side code can continue to stick to the C# ways of naming the things.
Adding a new customer
The click event handler of the Insert button is shown below:
$("#insert").click(function () {
var options = {};
options.url = "/api/customerservice";
options.type = "POST";
var obj = {};
obj.customerID = $("#newcustomerid").val();
obj.companyName = $("#companyname").val();
obj.contactName = $("#contactname").val();
obj.country = $("#country").val();
options.data = JSON.stringify(obj);
options.contentType = "application/json";
options.dataType = "html";
options.success = function (msg) {
$("#msg").html(msg);
};
options.error = function () {
$("#msg").html("Error while
calling the Web API!");
};
$.ajax(options);
});
The above code uses POST verb to make the Web API call. Moreover, it sets data, dataType and contentType properties. The data property is set to the stringified version of the new customer object. Notice that this new object also uses camel casing while setting the properties. The dataType property is set to html because our Post() action returns a plain string. The contentType property indicates the request's data type - JSON in this case.
The success function simply displays the message returned by the Post() action into the msg <div> element.
Modifying an existing customer
The click event of the Update button is shown below:
$("#update").click(function () {
var options = {};
options.url = "/api/customerservice/"
+ $("#customerid").val();
options.type = "PUT";
var obj = {};
obj.customerID = $("#customerid").val();
obj.companyName = $("#companyname").val();
obj.contactName = $("#contactname").val();
obj.country = $("#country").val();
options.data = JSON.stringify(obj);
options.contentType = "application/json";
options.dataType = "html";
options.success = function (msg) {
$("#msg").html(msg);
};
options.error = function (a, b, c) {
alert(c);
$("#msg").html("Error while
calling the Web API!");
};
$.ajax(options);
});
Most of the above code is similar to the code you wrote in the insert click event handler. The CustomerID being modified is appended to the url. The HTTP verb is set to PUT.
Deleting a customer
Finally, the code that deletes a customer is shown below:
$("#delete").click(function () {
var options = {};
options.url = "/api/customerservice/"
+ $("#customerid").val();
options.type = "DELETE";
options.dataType = "html";
options.success = function (msg) {
$("#msg").html(msg);
};
options.error = function () {
$("#msg").html("Error while
calling the Web API!");
};
$.ajax(options);
});
The above code sets the HTTP verb to DELETE and makes an Ajax call as before.
This completes the jQuery client to the Web API. Run the Index view and test all the operations.
In the next article you will develop a remote client to consume the Web API. Till then keep coding!!