Two trees A and B are quasi-isomorphic if A can be transformed into B by swapping left and right children of some of the nodes of A. The values in the nodes are not important in determining quasi-isomorphic trees, only the shape is important.
See the following two quasi-isomorphic trees.
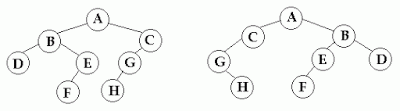
Now coming to your second question on construction of Quasi-Isomorphic Tree, since it is between two trees so we can write a function and passing two trees as parameters which can return two or false if quasi-isomorphic or not. Check the following code
struct node
{
int data;
struct node *lchild, *rchild;
}
bool is_quasi_isomorphic(struct node *treeA, struct node *treeB)
{
if (!treeA && !treeB) // Both are NULL so return true
return true;
if((!treeA && treeB) || (treeA && !treeB)) // Only one of them is null so false
return false;
return (is_quasi_isomorphic(treeA->lchild, treeB->lchild)
&& is_quasi_isomorphic(treeA->rchild, treeB->rchild)
|| is_quasi_isomorphic(treeA->rchild, treeB->lchild)
&& is_quasi_isomorphic(treeA->lchild, treeB->rchild));
}