Variable and Data Types in C#
A variable is an entity whose value can keep changing. For example, the age of a student, the age of a student, the address of a faculty member and the salary of an employee are all examples of variables.

In C#, a variable is a location in the computer’s memory that is identified by a unique name and is used to store a value. The name of the variable is used to access and read the value stored in it. Different types of data such as a character, an integer or a string can be stored in variables. Based on the type of data that needs to be stored in variable, variables can be assigned different data types.
Using variables
In C# , memory is allocated to a variable at the time of its creation. During creation, a variable is given a name that uniquely identifies the variable within its scope. For example, you can create a variable called empName to store the name of an employee.
You can initialize the variables at the time of creating the variable or at a later time. Once initialized, the value of a variable can be changed as required.
In c#, variable enable you to keep track of data. When you are referring to a variable, you are actually referring to the value stored in that variable.
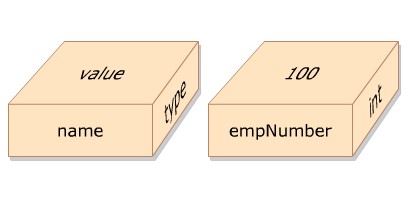
The following syntax is used to declare variable in c#,
<datatype> <variableName>;
Where,
Datatype: Is a valid data type in C#.
VariableName: Is a valid variable name.
Data Types
You can store different types of values such as numbers, characters or strings in different variables. But the compiler must know what kind of data a particular variable is expected to store. To identify the type of data that can be stored in a variable, c# provides different data types.
When a variable is declared, a data type is assigned to the variable. This allows the variable to store values of the assigned data types. In C# programming language, data types are divided into two categories. These are:
Value Types: variable of value types store actual values. These values are stored in a stack. The values can be either of a built-in data type or a user-defined data type. Most of the built-in data type are value types. The value type built-in data types are int, float, double, char and bool. Stack storage results in faster memory allocation to variables of value types.
Reference Type: Variables of reference type store the memory address of other variables in a heap. These values can either belong to a built-in data type or a user-defined data type. For example, string is a built-in data type which is a reference type. Most of the user-defined data types such as class are reference types.

Predefined Data Types
The predefined data types are referred to as basic data types in c#. These data types have a predefined range and size. The size of the data type helps the compiler to allocate memory space and the range helps the compiler to ensure that the value assigned is within the range of the variable’s data type.
The predefined data types in c# is given below:-
Data Type | Size | Range |
byte | Unsigned 8-bit integer | 0 to 255 |
short | Signed 16-bit integer | -32, 768 to 32,767 |
int | Signed 32-bit integer | -2,147,483,648 to 2,147,483,647 |
long | Signed 64-bit integer | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 32-bit floating point with 7 digits precisions | ±1.5e-45 to ±3.4e38 |
double | 64-bit floating point with 15-16 digits precision | ±5.oe-324 to ± 1.7e308 |
decimal | 128-bit floating point with 28-29 digits precision | ±1.0 x 10e-28 to ±7.9 x 10e28 |
char | Unicode 16-bit character | U+0000 to U+ffff |
bool | Stores either true or false | True or flase |