At first let’s see what different kind of buttons exist:
OK
OK, Cancel
Yes, No
Yes, No, Cancel
Retry, Cancel
Abort, Retry, Ignore
A message box is a dialog box that displays an alert or a message or also lets the user have some options to choose from.
A simple message box has an OK button
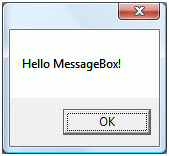
Simple MessageBox
A simple MessageBox shows a message and only has an OK button. Clicking on the OK button closes the MessageBox. The following line of code uses the Show method to display a message box with a simple message:
MessageBoxResult result = MessageBox.Show("Hello MessageBox");
The MessageBox generated by the line of code above is a modal dialog with an OK button

MessageBox with Title
A MessageBox can have a title. The first parameter of the Show method is a message string and second parameter is title string of the dialog. The following code snippet creates a MessageBox with a message and a title.
MessageBoxResult result = MessageBox.Show("Hello MessageBox", "Confirmation");
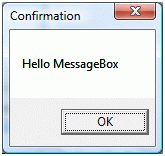
MessageBox with Title, Yes and No Buttons
The following code snippet creates a MessageBox with a message, a title, and two Yes and No buttons.
if (MessageBox.Show("Do you want to close this window?", "Confirmation", MessageBoxButton.YesNo) ==
MessageBoxResult.Yes)
{
// Close the window
}
else
{
// Do not close the window
}

MessageBox with Title, Yes, No and Cancel Buttons
The following code snippet creates a MessageBox with a message, a title, and two Yes, No, and Cancel buttons.
MessageBoxResult result = MessageBox.Show("Do you want to close this window?", "Confirmation",
MessageBoxButton.YesNoCancel);
if (result == MessageBoxResult.Yes)
{
// Yes code here
}
else if (result == MessageBoxResult.No)
{
// No code here
}
else
{
// Cancel code here
}

MessageBox with Title, Icon, Yes and No Buttons
A MessageBox also allows you to place an icon that represents the message and comes with some built-in icons. The MessageBoxImage enumeration represents an icon. Here is a list of MessageBoxImage enumeration values that represent the relative icons.
None
Hand
Question
Exclamation
Asterisk
Stop
Error
Warning
Information
The following code snippet creates a MessageBox with a message, a title, and two Yes and No buttons and an icon.
string message = "Are you sure?";
string caption = "Confirmation";
MessageBoxButton buttons = MessageBoxButton.YesNo;
MessageBoxImage icon = MessageBoxImage.Question;
if (MessageBox.Show(message, caption, buttons, icon) == MessageBoxResult.OK)
{
// OK code here
}
else
{
// Cancel code here
}
